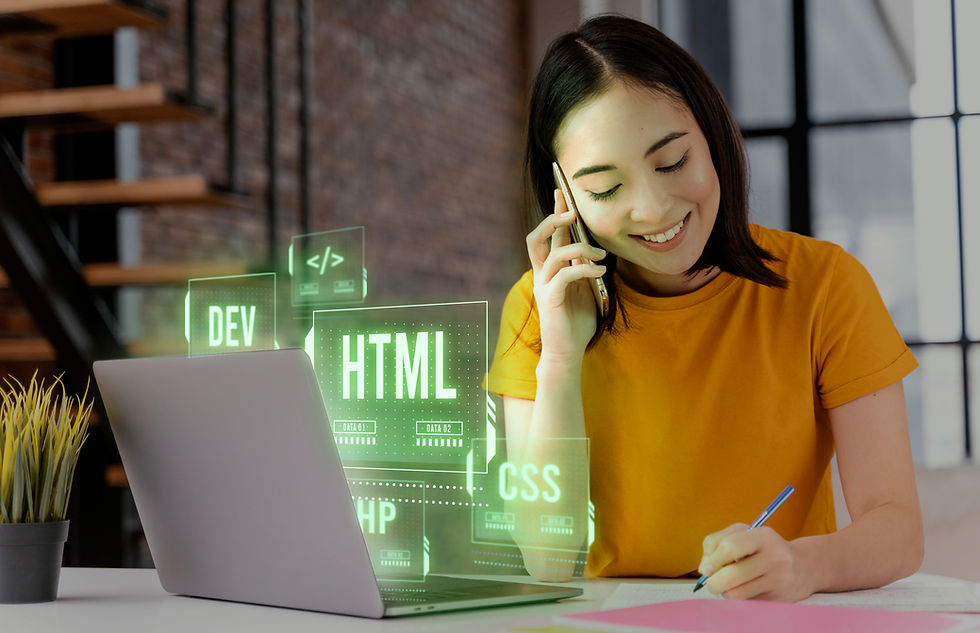
Creating a web application can be a daunting task, especially if you are new to the backend world. Node.js and Express.js provide a robust platform to build efficient and scalable web applications. In this tutorial, we will walk you through the process of building your very first web application using these technologies.
What Are Node.js and Express.js?
Before we dive in, let's briefly discuss what Node.js and Express.js are:
Node.js is a runtime environment that allows you to run JavaScript on the server side. It's built on Chrome's V8 JavaScript engine, and it's known for its efficiency and scalability.
Express.js, or simply Express, is a web application framework for Node.js. It's designed for building web applications and APIs. It has been called the de facto standard server framework for Node.js.
Prerequisites
To follow this tutorial, you should have the following installed on your machine:
Node.js
NPM (Node Package Manager), which comes with Node.js
A text editor (like Visual Studio Code, Atom, Sublime Text, etc.)
A basic understanding of JavaScript
Step 1: Setting Up Your Project
First, create a new directory for your project and navigate into it:
shellCopy code
mkdir my-first-node-app cd my-first-node-app
Initialize a new Node.js project:
shellCopy code
npm init -y
The -y flag will automatically fill in the defaults in the package.json file for you.
Step 2: Installing Express
Install Express using NPM:
shellCopy code
npm install express --save
The --save flag will add Express as a dependency in your package.json file.
Step 3: Creating Your First Server
Create a file named app.js in your project directory:
shellCopy code
touch app.js
Open app.js in your text editor and start by requiring Express:
javascriptCopy code
const express = require('express'); const app = express();
Set up a basic route to serve an HTTP GET request on the root path:
javascriptCopy code
app.get('/', (req, res) => { res.send('Hello World!'); });
Tell your app to listen on a port:
javascriptCopy code
const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
Step 4: Running Your Server
Run your application:
shellCopy code
node app.js
Open your web browser and navigate to http://localhost:3000. You should see "Hello World!" displayed.
Step 5: Adding More Functionality
Let’s expand your application by adding more routes. For example, create an about page:
javascriptCopy code
app.get('/about', (req, res) => { res.send('About this web application'); });
Restart your server and visit http://localhost:3000/about to see the new page.
Step 6: Using Templates
While sending text is fine, most web applications use templates to render HTML. Install a templating engine like ejs:
shellCopy code
npm install ejs --save
Configure Express to use EJS:
javascriptCopy code
app.set('view engine', 'ejs');
Create a views directory in your project folder and add an index.ejs file with some HTML content.
Update your root route to render the index.ejs file:
javascriptCopy code
app.get('/', (req, res) => { res.render('index'); });
Step 7: Middleware
Express is all about middleware. Add some basic middleware to serve static files from a public directory:
javascriptCopy code
app.use(express.static('public'));
Now, any file in the public directory will be served by Express.
Conclusion
Congratulations! You’ve just created a basic web application with Node.js and Express. This is just the starting point of your journey into web development. From here, you can learn about connecting to databases, user authentication, and more complex routing and business logic.
Remember, the key to becoming proficient in web development is practice and continual learning. Explore the vast ecosystem of Node.js and Express, and you'll be building sophisticated applications in no time. Happy coding!
Commentaires